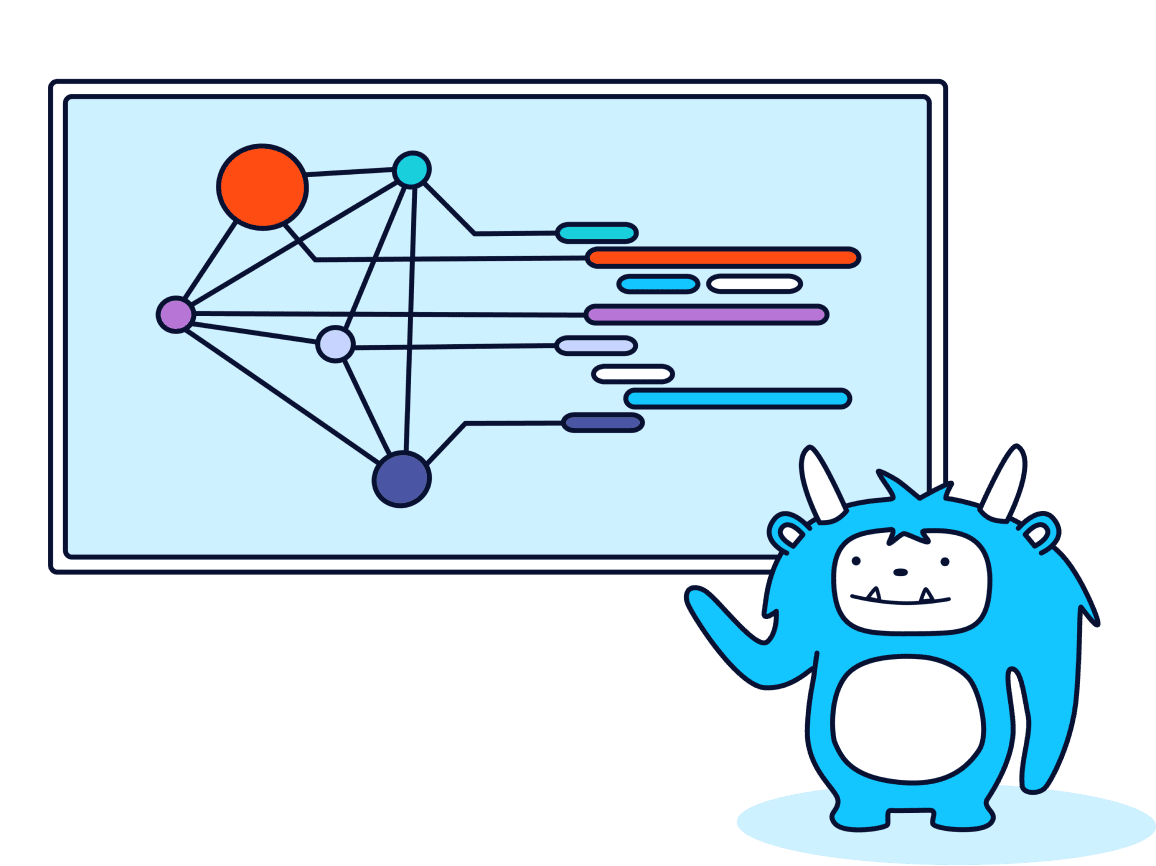
Learn
Fluree Overview
What sets Fluree apart and how it can help you
Tutorial
Learn the essentials of using Fluree and develop an accurate mental model of the system
Guides
Get into the details of working with Open Standards and Fluree
Foundations
Deep dives into slices of Fluree functionality
Reference
Fluree HTTP API
The basics of transacting, query, and creating ledgers over HTTP
Cookbook
Examples of how to accomplish a comprehensive set of basic use cases
Error Codes
Lookup guide for each Fluree error code with links to supporting documentation